Hey there, fellow developers and API enthusiasts. If you’ve ever found yourself scratching your head over understanding the difference between PUT, POST, and PATCH in RESTful API, you’re not alone.
These HTTP methods might seem similar at first glance, but they serve distinct purposes. As a software engineer who’s wrestled with these concepts in real world projects, I’m here to break it down for you with clear explanations, practical examples to bring it all to life. Let’s dive in.
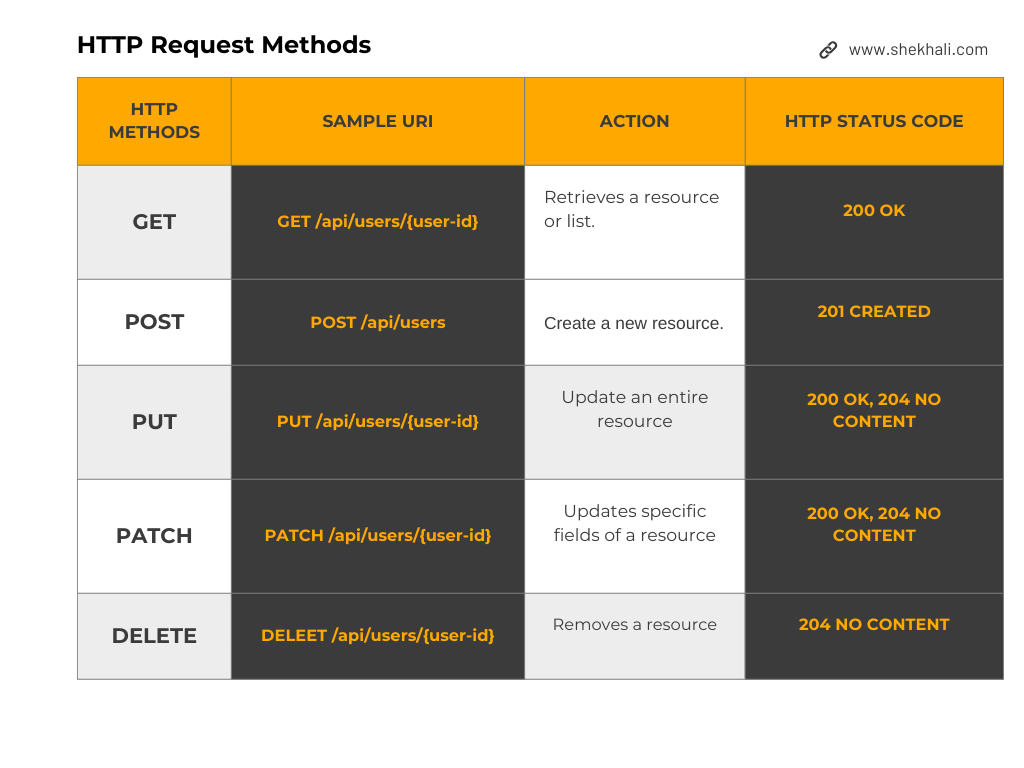
Table of Contents
- 1 What Are REST APIs, Anyway?
- 2 What Are HTTP Methods?
- 3 POST (Create a New Resource)
- 4 PUT (Full Update or Replace Resource)
- 5 PATCH (Partial Update)
- 6 When to Use POST, PUT, and PATCH?
- 7 Frequently Asked Questions (FAQs)
- 7.1 Q: Can I use HTTP PUT method to create a resource?
- 7.2 Q: What’s the difference between idempotent and non-idempotent?
- 7.3 Q: Difference between PUT PATCH POST in REST API methods?
- 7.4 Q: Should I use PATCH or PUT for updating passwords?
- 7.5 Q: What happens if I send a PUT request without all fields?
- 7.6 Q: Is PATCH always idempotent?
- 7.7 Related
What Are REST APIs, Anyway?
Before we get into the nitty-gritty, let’s set the stage. REST (Representational State Transfer) is an architectural style for designing networked applications.
It relies on HTTP methods like GET, POST, PUT, PATCH, and DELETE to perform CRUD operations (Create, Read, Update, and Delete) on resources (think users, products, etc.).
Today, we’re focusing on three key players: POST, PUT, and PATCH. Each has its own superpower, and knowing when to use them can make your API clean, efficient, and intuitive.
What Are HTTP Methods?
Alright, let’s kick things off with the basics. HTTP methods define actions that can be performed on a resource, they tell a server what you want to do with a resource. The most commonly used ones are:
- GET → The GET method retrieve data (example, Fetch a user list).
- POST → The POST method Create a new resource (example, Add a new user).
- PUT → The PUT method update or replace an entire resource.
- PATCH → The PATCH method Partially update a resource.
- DELETE → The DELETE method remove a resource.
Among these, POST, PUT, and PATCH are crucial for data modification. Let’s explore them in detail, trust me, it’s worth the ride.
POST (Create a New Resource)
The POST method is used to create a new resource. Every time you send a POST request, a new record is created.
Let’s Imagine you’re Submitting a form to register a new user. You send a POST request with the data (name, email, age), and the server creates a new resource, usually returning a HTTP status code 201 Created
and the resource’s ID or URI like http://localhost:5000/api/users
.
Key Points About POST:
✅ Purpose: The POST method is used to create new resources.
✅ It does not replace or update existing resources.
✅ The server generates a new unique ID for each resource.
✅ Not idempotent: sending the same POST request multiple times creates multiple resources..
Example: Creating a User
📌 Request (POST /api/users)
POST /api/users
Content-Type: application/json
{
"name": "Shekh Ali",
"email": "shekh.ali@example.com",
"age": 25,
"status": "Single"
}
📌 Response
HTTP/1.1 201 Created
Location: /api/users/1
{
"id": 1,
"name": "Shekh Ali",
"email": "shekh.ali@example.com",
"age": 25,
"status": "Single"
}
PUT (Full Update or Replace Resource)
The PUT method is used to update an existing resource by replacing the entire object.
You send the entire resource data, and the server updates it completely. Think of PUT as swapping out an old file with a new one. Successful updates return a HTTP status code 200 OK
(with updated data) or 204 No Content
(no data returned), while a successful creation return a HTTP status code 201 Created
.
Key Points About PUT
✅ Purpose: The PUT method is used to update an existing resource. If the resource does not exist, it may create a new one.
✅ PUT Replaces the entire object, not just a part of it.
✅ Idempotent: It is idempotent since sending the same PUT request multiple times results in the same outcome.
✅ Example: We can use PUT when Updating a user’s full profile (name, email, age, phone, etc.).
Example: Updating a User with PUT
Let’s say USER needs a full update because the age and status changed:
📌 Request (PUT /api/users/1)
PUT /api/users/1
Content-Type: application/json
{
"id": 1,
"name": "Shekh Ali",
"email": "shekh.ali@example.com",
"age": 26,
"status": "Married"
}
Response:
HTTP/1.1 200 OK
{
"id": 1,
"name": "Shekh Ali",
"email": "shekh.ali@example.com",
"age": 26,
"status": "Married"
}
PATCH (Partial Update)
The PATCH method is used to update only specific fields of a resource without affecting others. It is used for partially updating a resource. Instead of sending the whole object, you only send the fields you want to change. It’s like editing a single line in a document rather than rewriting the whole thing.
Key Points About PATCH:
✅ Purpose: The PATCH method is used for partial updates. It Does not replace the entire resource, only updates specified fields.
✅ Idempotent (mostly): sending the same request multiple times results in the same resource update.
✅ Example: Changing just a user’s age or email address.
Example: Updating Only Age with PATCH
Now, let’s say we only want to update user’s age without touching the other fields:
📌 Request (PATCH /api/users/1)
PATCH /api/users/1
Content-Type: application/json
{
"age": 27
}
Response:
HTTP/1.1 200 OK
{
"id": 1,
"name": "Shekh Ali",
"email": "shekh.ali@example.com",
"age": 27,
"status": "Married"
}
See the difference? POST creates, PUT replaces everything, and PATCH tweaks just what you need.
When to Use POST, PUT, and PATCH?
✔️ Use POST when creating a new resource, such as adding a new user, product, or order where the server assigns the identifier (e.g., a new URI or ID). It’s your go-to for fresh data creation.
✔️ Use PUT when completely updating or replacing an existing resource. It’s ideal when you’re updating an entire entity like a user profile.
✔️ Use PATCH when updating only specific fields of a resource (e.g., a user’s email) while leaving the rest untouched. It’s efficient for partial changes without a full rewrite.
Frequently Asked Questions (FAQs)
Q: Can I use HTTP PUT method to create a resource?
Technically, yes, you can use PUT to create a resource but it’s not RESTful. HTTP PUT method is meant to update a resource at a specific URI. If the resource doesn’t exist, some APIs create it, but POST is the standard for creation.
Q: What’s the difference between idempotent and non-idempotent?
An idempotent method (like PUT) produces the same result no matter how many times you call it. A non-idempotent method (like POST) can create new outcomes with each call.
Q: Difference between PUT PATCH POST in REST API methods?
Use POST to create a new resource (e.g., a new user). Choose PUT to fully replace an existing resource. Pick PATCH to partially update just a few fields like changing an email without touching the rest.
Q: Should I use PATCH or PUT for updating passwords?
I’d preferred PATCH
here, as it updates only the password field without affecting other user details.
A password update usually involves just one field-why send the whole user object with PUT when you can send {"password": "newsecret"}
with PATCH? It’s lighter and fits the “partial update” vibe.
Q: What happens if I send a PUT request without all fields?
Here’s the catch with HTTP PUT
: it’s a full replacement. If you skip fields in your payload, the server might set those missing ones to null or their default values, wiping out what was there before. For example, updating a user with just {"name": "Sheikh Ali"}
could erase their email or role if the API doesn’t preserve them. Always send the complete resource or use PATCH
for partial changes.
Q: Is PATCH always idempotent?
Nope, it’s not a guarantee. Idempotency means repeat calls give the same result like PUT does. With PATCH, it depends on how you use it. Setting a field to a fixed value (e.g., “status”: “done”) can be idempotent- do it ten times, same outcome. But if you’re incrementing something (e.g., “likes”: +1″), each call changes the state, so it’s not. It’s up to your design, so plan carefully.
References:
Recommended Articles:
- What is Web API? Everything You Need to Learn
- Web services vs API: Top 10+ difference between API and Web Service
- C# dispose vs finalize – Understanding the difference between dispose and finalize in C#
- IEnumerable VS IQueryable with code examples
- C++ vs C#: Difference Between C# and C++
- C# Abstract class Vs Interface
- WCF vs Web API: Top 10 Differences Between WCF and Web API
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025