In this article, we’ll explore how to write a C# program to check if a Given Number is Even or Odd.
C# program to count vowels and consonants in a string
In this article, we’ll discuss how to count vowels and consonants in a string in the C# program.
Method1: C# program to count vowels and consonants in a string
Let’s start with a simple C# program that count vowels and consonants in a string. Below is the complete code, and we’ll break it down step by step.
How to remove special characters from a string in C#
Understanding the Challenge
Before diving into the code, let’s grasp the concept of special characters. Special characters are those that fall outside the realm of alphanumeric characters.
They include symbols like '@', '#', '$', '%'
, and more. The goal is to create a C# program that efficiently removes these special characters from a given string and allowed characters are A-Z (uppercase or lowercase) and numbers (0-9).
C# program to count the occurrences of each character in a String
Understanding the Problem:
Before we start writing code, it’s crucial to understand the problem at hand.
We want to create a C# program that takes a string as input and produces a count of each unique character in that string.
How to Remove Duplicate Characters from a String in C#: A Beginner’s Guide
In this article, we’ll learn how to remove duplicate characters from a String in C# using multiple examples.
Exploring HashSet in C# with Examples
In this article, we will take a deep dive into HashSet in C# with real world examples and clear explanations along the way.
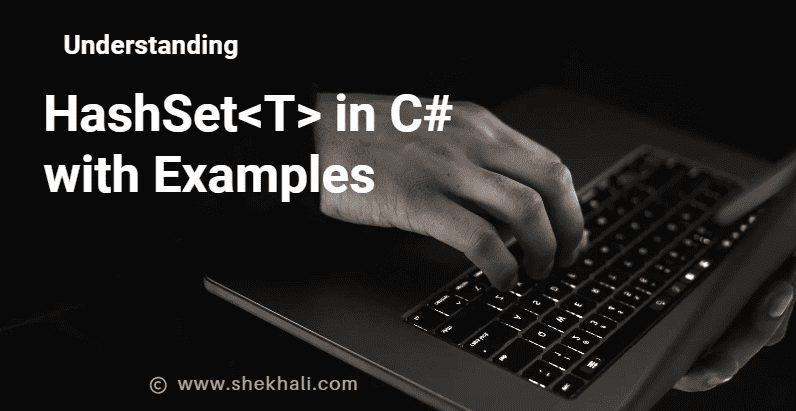
Understanding List vs Dictionary in C# With Examples
When it comes to working with collections in C#, you’ll often find yourself deciding between using a List or a Dictionary, as both serve for storing and managing data and belong to System.Collection.Generics
namespace.
In this article, we’ll discuss List vs Dictionary in C# with Examples and will understand when to use each and why.
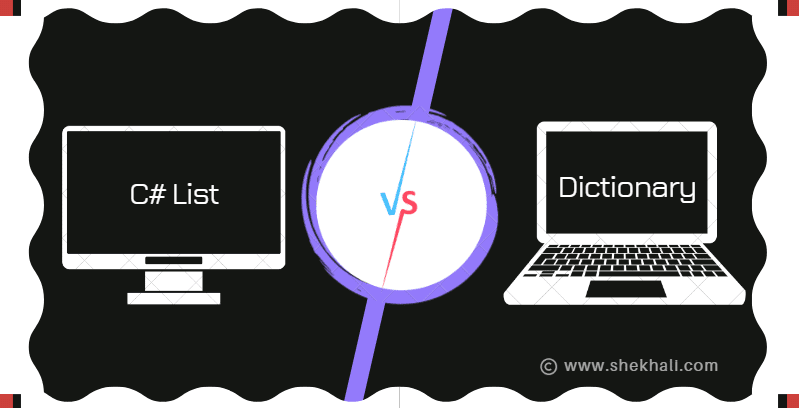
Understanding the Difference Between Overriding and Overloading in C#
Overriding and Overloading the two important concepts of Polymorphism.Â
Overloading in C# is known as Static Polymorphism or Compile time Polymorphism. In Overloading we can create methods with the same name but different parameters within the same class.
On the other hand, Overriding is known as dynamic Polymorphism or Runtime Polymorphism which allows us to provide different implementation of a method in Inherited Classes.
In this article, we will learn the differences between overriding and overloading in C# with practical code examples.
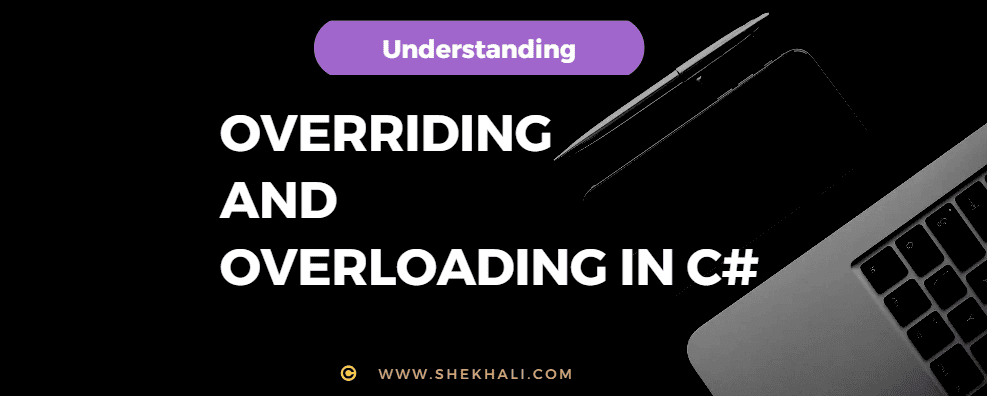
CTE in SQL Server: Learn Common Table Expressions with Examples
CTE in SQL simplifies complex queries to make code more readable and provides a structured way to work with temporary result sets.
In this article, we will explore what CTE is, its syntax, examples, why we need it, its types, advantages, and disadvantages of Common Table Expression (CTE), and how to use it in SQL Server.
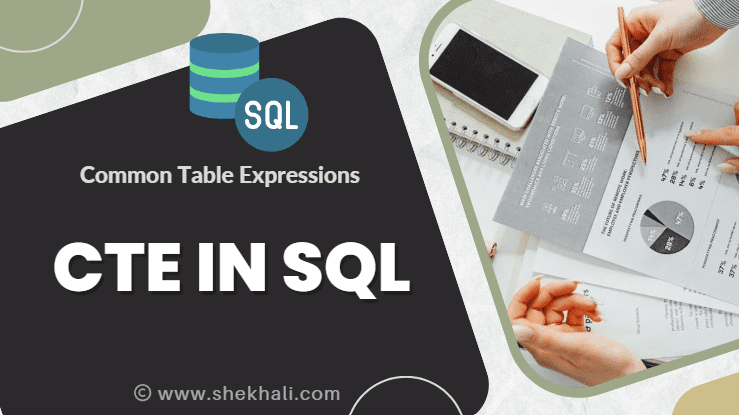
Static vs Singleton in C#: Understanding the Key Differences
In this article, we will explore the Static vs Singleton in C# with code examples and detailed explanations.
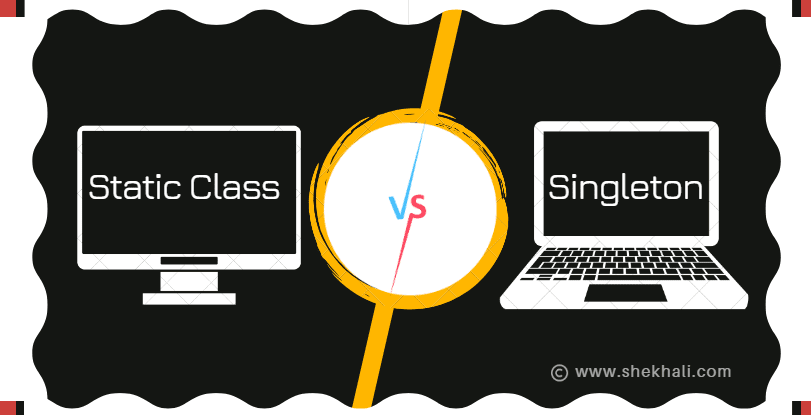
SOLID Principles – Dependency Inversion Principle in C# With Code Examples
In this article, we’ll learn the Dependency Inversion Principle in C# with code examples. We will explore its significance, benefits, and implementation using real-world examples.
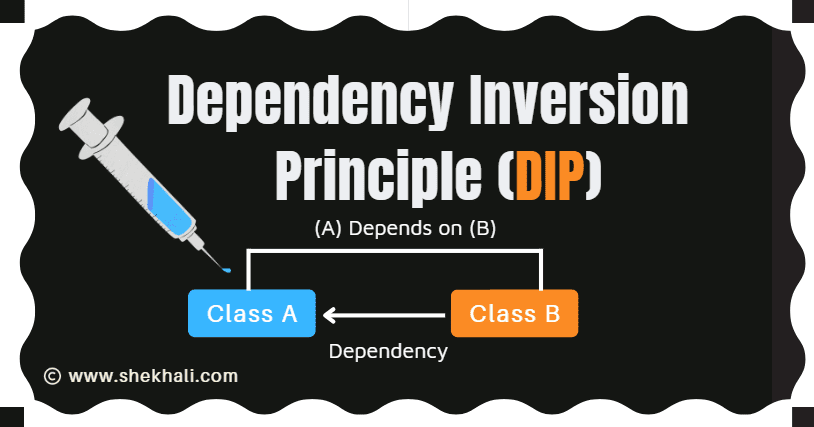
Understanding the C# Interface Segregation Principle (ISP) with Examples
In this article, we will learn about the C# Interface Segregation Principle (ISP), which states that a class should not forced to implement Interface methods they don’t use.
It promotes the creation of focused, smaller, and modular interfaces to prevent clients from implementing methods they don’t need.
We will learn its significance and how it can be applied using C# code examples.
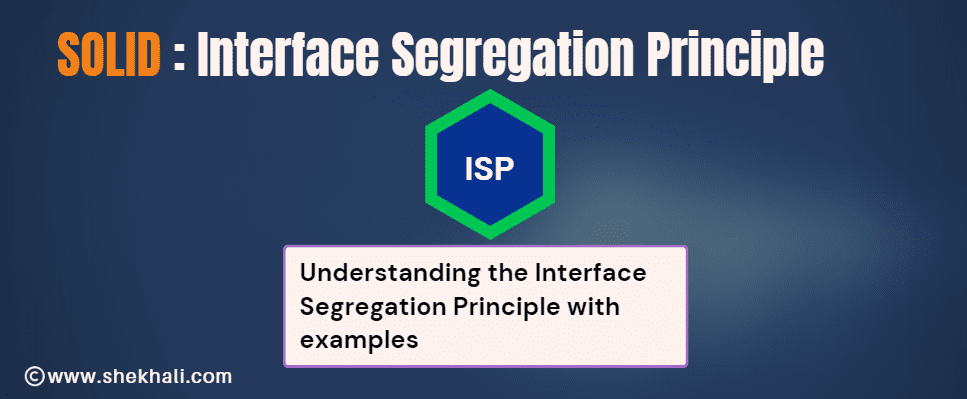
C# Liskov Substitution Principle With Examples
In this article, we will learn the C# Liskov Substitution Principle and understand implementation to build scalable and easier to maintain software applications.
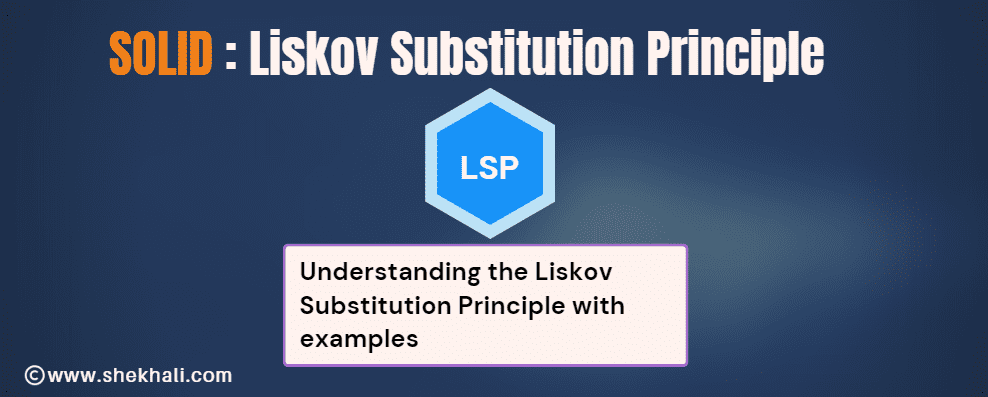
Understanding Covariance and Contravariance in C# – A Comprehensive Guide
The concepts of Covariance and Contravariance in C# might initially sound complex, but fear not! By the end of this article, you’ll have a crystal-clear understanding of how they work and how to leverage them in your C# programming.
“Covariance and Contravariance are terms used in programming languages to describe how subtypes relate to their base types. Covariance is when a derived type can be used where a base type is expected. On the other hand, Contravariance is when a base type can be used where a derived type is expected.“
Covariance and contravariance deal with how type conversions are allowed between reference types in C#. These concepts come into play when working with arrays, delegates, and interfaces.
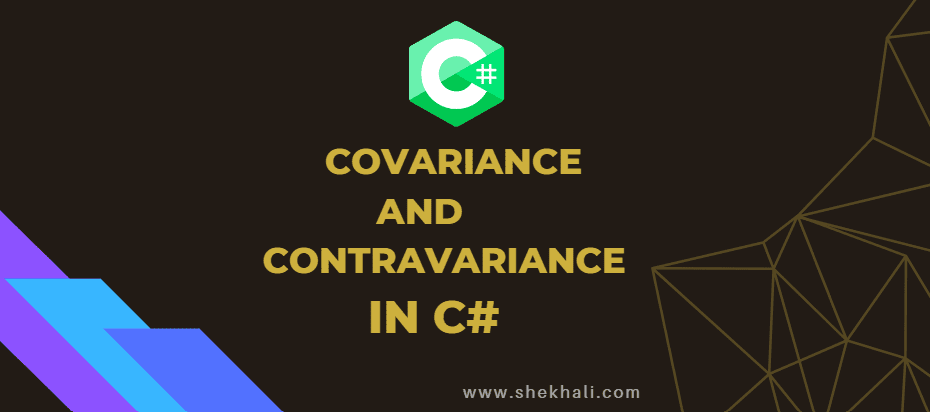
Top 50+ JavaScript Interview Questions & Answers (2024)
Here, we have a list of 50 + commonly asked JavaScript interview questions, simplified answers, and code examples. It will help you prepare for your interview. Let’s get started:
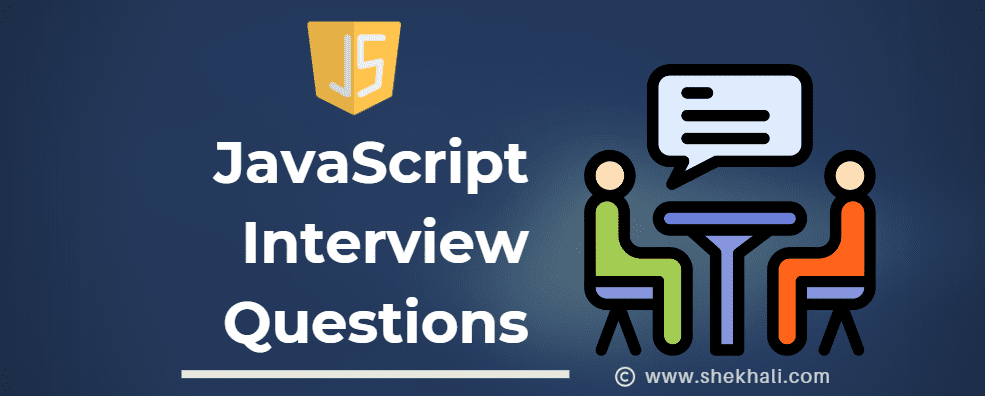
Understanding 3-Tier Architecture in C#: A Comprehensive Guide with Examples
In software development, designing modular and maintainable applications is very important. This is where architectural patterns come into play. One such popular pattern is the 3-Tier Architecture, which provides a structured approach to organizing code in separate layers.
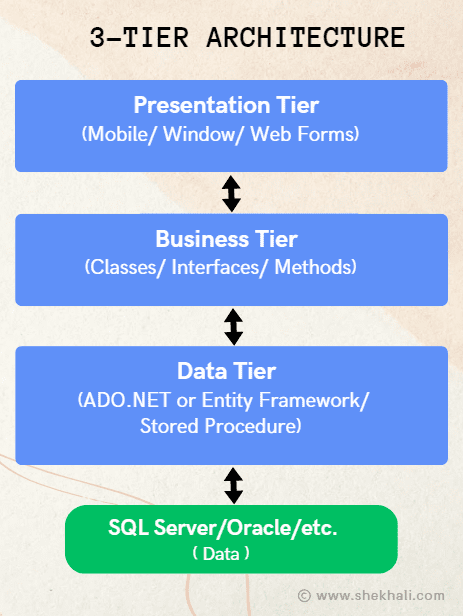
Understanding C# Abstract Class With Examples
An abstract class in C# serves as a blueprint for other classes. It cannot be instantiated on its own and is meant to be a base class of other classes. It may contain both abstract and non-abstract members (methods, properties, or events). The abstract members must be implemented by non-abstract derived classes.
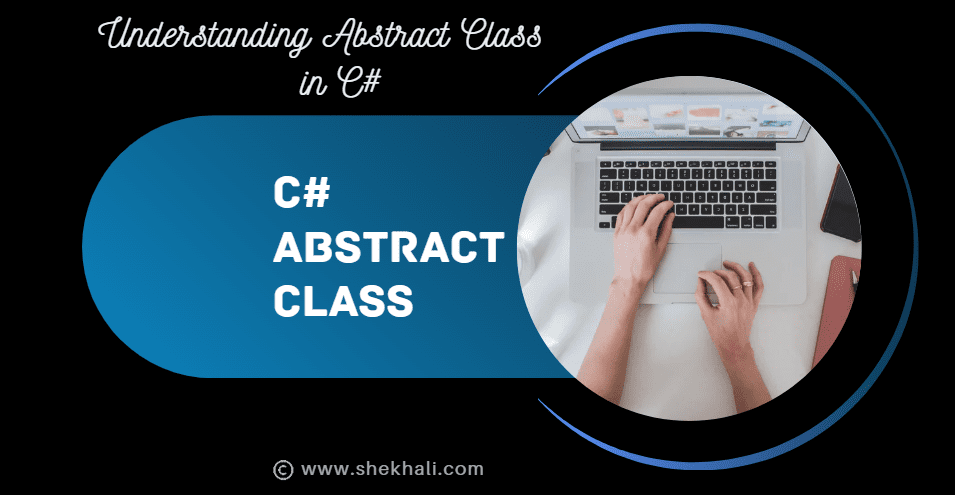
Understanding C# Class and Objects
In C# programming, class and objects are essential building blocks of object-oriented programming. Objects in C# are like real-world things we can touch or interact with, and classes are like plans or templates to create these objects.Â
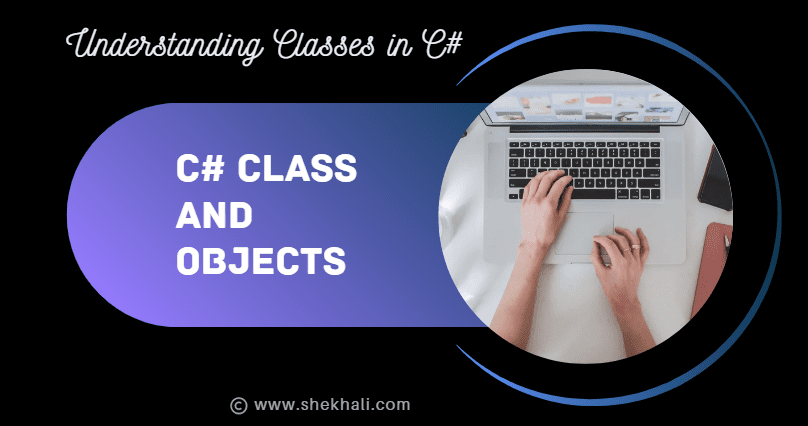
C# do while Loop: A Beginner’s Guide
In C# programming, the do while loop
is a control flow statement that allows you to execute a code block based on a condition repeatedly.Â
In this article, we will explore the C# do while loop, its syntax, and working principle and provide practical examples to help students and professionals to grasp its concepts easily.