As a software engineer, you’ve likely encountered scenarios where you need to build services to connect applications or expose functionality over the web. Two popular Microsoft technologies for this are Windows Communication Foundation (WCF) and ASP.NET Web API. But what’s the real difference between WCF and Web API?
When should you use one over the other? In this article, I’ll break it down for you with examples, a comparison table, and practical insights straight from my experience as a developer.
Let’s dive in and explore these technologies step by step.
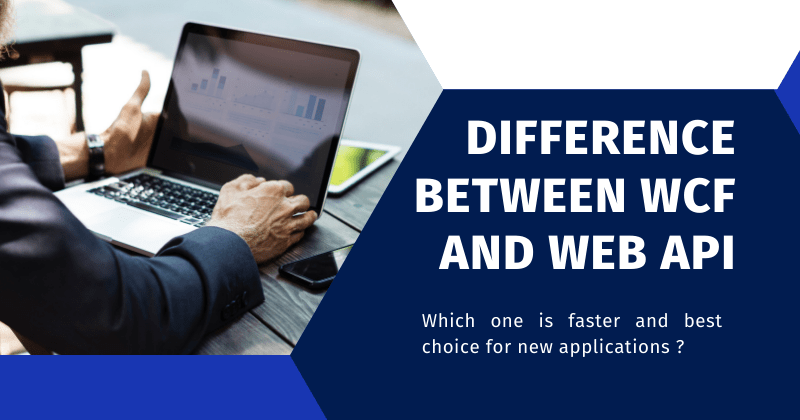
Table of Contents
- 1 What is WCF?
- 2 📌Key Features of WCF:
- 3 What is Web API?
- 4 📌Key Features of Web API:
- 5 WCF vs Web API: A Side-by-Side Comparison
- 6 When to Use WCF vs Web API?
- 7 When to Use Web API?
- 8 Key Differences in Code: WCF vs Web API
- 9 Which One Should You Choose?
- 10 FAQs About WCF and Web API:
- 10.1 Q: What is the main difference between WCF and Web API?
- 10.2 Q: Can Web API replace WCF?
- 10.3 Q: Is WCF outdated in 2025?
- 10.4 Q: Which is faster: WCF or Web API?
- 10.5 Q: Which one is more secure WCF or Web API?
- 10.6 Q: What is the best alternative to WCF for new applications?
- 10.7 Q: Does Web API support duplex communication?
- 11 Conclusion:
What is WCF?
Windows Communication Foundation (WCF) was introduced by Microsoft in .NET Framework 3.0, is a robust framework for building service oriented applications. It’s designed to enable communication between applications across different platforms using various protocols like HTTP, TCP, MSMQ, and Named Pipes.
Think of WCF as a Swiss Army knife, it’s versatile and can handle everything from SOAP-based services to complex enterprise systems.
📌Key Features of WCF:
✅ WCF Supports multiple communication protocols (HTTP, TCP, Named Pipes, MSMQ, etc.).
✅ WCF has Built-in support for SOAP (Simple Object Access Protocol) and WS-* standards (e.g., WS-Security, WS-ReliableMessaging
).
✅ WCF Can be hosted in IIS, Windows Services, or self-hosted in an application. To consume a WCF service, a client needs to generate a proxy using svcutil.exe
✅ It Provides security, transaction management, and reliability features. So, It Ideal for enterprise-level, secure, and transactional services.
✅ Supports duplex communication.
✅ Configurable via code or XML configuration files.
What is Web API?
ASP.NET Web API was introduced later with .NET Framework 4.0, is a lightweight framework for building HTTP-based RESTful services. It’s easy to use and perfect for modern web and mobile applications that need to expose data in formats like JSON or XML.
If you’re building an API for a single-page application (SPA) or a mobile client then Web API is often your go to choice.
📌Key Features of Web API:
✅ Web API Supports RESTful services using HTTP protocol. It is Lightweight and easy to use for modern web & mobile apps.
✅ Web API Supports JSON, XML and other content types out of the box.
✅ It integrates effortlessly with ASP.NET MVC and provides robust authentication and authorization options, including OAuth and JWT.
✅ Web APIs are typically hosted in IIS or a self-hosted environment using Kestrel. They can be consumed using HTTP clients like Postman, Fetch API, or HttpClient
in C#.
✅ Web API Supports Attribute routing which offers greater control and flexibility over URL configurations.
WCF vs Web API: A Side-by-Side Comparison
To make things clearer, let’s compare the differences between WCF and Web API in a tabular format:
Feature | WCF (Windows Communication Foundation) | Web API |
---|---|---|
Protocols Supported: | WCF supports SOAP, HTTP, TCP, MSMQ, Named Pipes. | Web API supports only HTTP. it’s perfect for modern apps that live in the cloud or talk to browsers and mobile devices. |
Message Format: | XML (SOAP-based messages) | JSON, XML (default JSON) |
Hosting: | WCF can be hosted in IIS, self-hosted, Windows Services | Web API sticks to IIS or self-hosting (like with OWIN or Kestrel in .NET Core). |
Best for: | WCF is best for Enterprise applications needing SOAP-based services, legacy systems, or scenarios needing transactions and reliability (e.g., banking systems). | Web API is best for RESTful services, web & mobile applications. It is light weight, modern and developer friendly. |
Security: | It supports WS-Security, Transport security, Message security | Web API supports OAuth, JWT, Basic Authentication |
Performance: | WCF is slightly slower due to SOAP overhead. All that XML parsing and protocol complexity slows it down, but it’s a trade off for its robustness in enterprise use cases. | Web API is faster due to lightweight RESTful nature. it outperform WCF in real time apps where every millisecond counts. |
Support for REST: | WCF can do REST, but it’s a hassle as you need WebHttpBinding and extra configuration. | Web API has built-in support for RESTful services means you’re writing GET, POST, and DELETE endpoints in minutes—no extra hoops to jump through. |
Complexity: | WCF is more complex. The endless configuration options (bindings, endpoints, behaviors) can overwhelm you. I’ve spent hours tweaking App.config files. It’s powerful, but not fun. | Web API is simpler and easy to implement. You’re coding controllers and routes, not drowning in XML. It’s what I’d recommend to anyone starting out. |
This table highlights why Web API is often preferred for modern development, while WCF still shines in legacy or enterprise scenarios.
When to Use WCF vs Web API?
✔️ Use WCF when you need to support multiple protocols (e.g., HTTP, TCP, MSMQ, etc.).
✔️ Use WCF when your application requires SOAP-based messaging with WS-* standards.
✔️ Use WCF when you are working on a legacy or distributed enterprise application with complex security or transaction needs.
✔️ Use WCF when you need duplex communication (e.g., two-way messaging).
WCF Example: Creating a Simple Service
Let’s create a basic WCF service to calculate the sum of two numbers.
- Define the Service Contract:
using System.ServiceModel;
[ServiceContract]
public interface ICalculatorService
{
[OperationContract]
int Add(int a, int b);
}
2. Implement the Service:
public class CalculatorService : ICalculatorService
{
public int Add(int a, int b)
{
return a + b;
}
}
3. Host the Service (e.g., in a console app):
using System.ServiceModel;
class Program
{
static void Main()
{
using (ServiceHost host = new ServiceHost(typeof(CalculatorService)))
{
host.Open();
Console.WriteLine("WCF Service is running...");
Console.ReadLine();
}
}
}
4. Configuration (in App.config):
<system.serviceModel>
<services>
<service name="CalculatorService">
<endpoint address="http://localhost:8000/Calculator"
binding="basicHttpBinding"
contract="ICalculatorService"/>
</service>
</services>
</system.serviceModel>
This service can now be consumed by a client over HTTP using SOAP. Pretty powerful, right? But it’s also a bit heavy for simple tasks.
When to Use Web API?
✔️ Use Web API when you’re building RESTful services for web or mobile clients.
✔️ Use Web API when you need lightweight, fast communication with JSON/XML.
✔️ Use Web API when you’re working with modern frameworks like .NET Core and you need better performance and scalability.
Web API Example: Creating a RESTful Endpoint
Let’s build a simple Web API to perform the same addition operation.
- Create a Controller:
using Microsoft.AspNetCore.Mvc;
[Route("api/[controller]")]
[ApiController]
public class CalculatorController : ControllerBase
{
[HttpGet("add")]
public IActionResult Add(int a, int b)
{
var result = a + b;
return Ok(new { Result = result });
}
}
2. Run the API:
- Host it in an ASP.NET Core app (e.g., via IIS or Kestrel).
- Access it at the URL for example:
http://localhost:5000/api/calculator/add?a=5&b=3
.
📌 3. Response (in JSON):
{
"Result": 8
}
See how simple that was? No complex configuration but just pure HTTP and JSON.
Key Differences in Code: WCF vs Web API
- WCF: Relies on service contracts, endpoints, and bindings. It’s verbose but flexible.
- Web API: Uses controllers and HTTP verbs (GET, POST, PUT, DELETE etc.). It’s concise and REST-friendly.
From a developer’s perspective, Web API feels more intuitive for quick projects also it is lightweight, fast and easy to integrate with modern apps. While, WCF offers deeper control for enterprise needs with Strong security and transaction support also it is Ideal for legacy systems.
Which One Should You Choose?
Here’s my take: If you’re starting a new project in 2025, Web API (or its evolution in ASP.NET Core) is usually the way to go. It aligns with modern development trends. Just think microservices, cloud-native apps, and mobile client integrations, offering a lightweight, flexible, and future ready solution.
On the other hand, WCF remains relevant if you’re working with legacy systems or need advanced protocol support beyond HTTP (like TCP or MSMQ), or need enterprise grade features such as distributed transactions and advanced security configurations.
FAQs About WCF and Web API:
Q: What is the main difference between WCF and Web API?
The core difference is their purpose: WCF (Windows Communication Foundation) is a versatile framework for building service-oriented apps, supporting multiple protocols like HTTP, TCP, and MSMQ, and relying heavily on SOAP and XML.
Web API, on the other hand, is a lightweight, HTTP-only framework focused on RESTful services, delivering data in JSON or XML. Think of it as a streamlined option for modern web and mobile apps. In short, WCF is broad and complex whereas Web API is simple and web centric.
Q: Can Web API replace WCF?
Not entirely, it depends on your needs. Web API can replace WCF for HTTP-based, RESTful services, like APIs for web or mobile apps, because it’s faster and easier to use. I’ve swapped WCF for Web API in projects needing JSON over HTTP and never looked back.
But if you’re using WCF’s unique features like TCP, MSMQ, or SOAP with WS-Security then Web API won’t cut it. So, for web-focused projects I would say yes but for enterprise multi protocol setups definitely no.
Q: Is WCF outdated in 2025?
Not outdated, but it’s definitely past its prime. As of 2025, WCF still has a place in legacy enterprise systems where SOAP, transactions, or non-HTTP protocols are critical for example banking or old-school integrations. But for new projects, especially with .NET Core (where WCF support is limited), Web API or newer options like gRPC are stealing the spotlight. It’s not dead, just less trendy.
Q: Which is faster: WCF or Web API?
Web API is generally faster and wins the speed race because it uses lightweight RESTful architecture without SOAP overhead. It’s perfect for quick responses in web or mobile apps.
On the other hand, WCF with its SOAP and XML baggage is slower because of the extra processing and larger message sizes. It’s built for robustness and not for raw speed, so choose based on whether performance or features matter more to you.
Q: Which one is more secure WCF or Web API?
Both WCF and Web API support security, but WCF offers WS-Security, transport, and message security, whereas Web API uses OAuth, JWT, and HTTPS.
Q: What is the best alternative to WCF for new applications?
For new applications, ASP.NET Core Web API and gRPC are recommended over WCF.
Q: Does Web API support duplex communication?
No, Web API is stateless and does not support duplex communication, while WCF does.
Conclusion:
WCF and Web API are like two sides of the .NET coin, each shines in its own arena. Both WCF and Web API serve different purposes in .NET applications.
WCF is ideal for enterprise-level SOAP-based services and can be useful in scenarios demanding multi-protocol support (HTTP, TCP, MSMQ) and advanced features like WS-Security or transactional integrity, while Web API is best for RESTful, lightweight, and modern web-based applications. Choosing between the two depends on your project requirements.
References:
- REST API Tutorial
- Learn WCF, Web API, WCF REST and Web Service (stackoverflow)
Recommended Articles:
- What is Web API? Everything You Need to Learn
- Web services vs API: Top 10+ difference between API and Web Service
- PUT vs PATCH vs POST in REST API
- C# 12 New Features in 2025: What’s New with .NET 8 - April 11, 2025
- Difference Between WCF and Web API with Examples: A Comprehensive Guide - March 27, 2025
- PUT vs PATCH vs POST in REST API: Key Differences Explained With Examples - March 23, 2025