In this article, we will learn the basic difference between array and ArrayList in C#. It will help you make informed decisions for your C# projects.
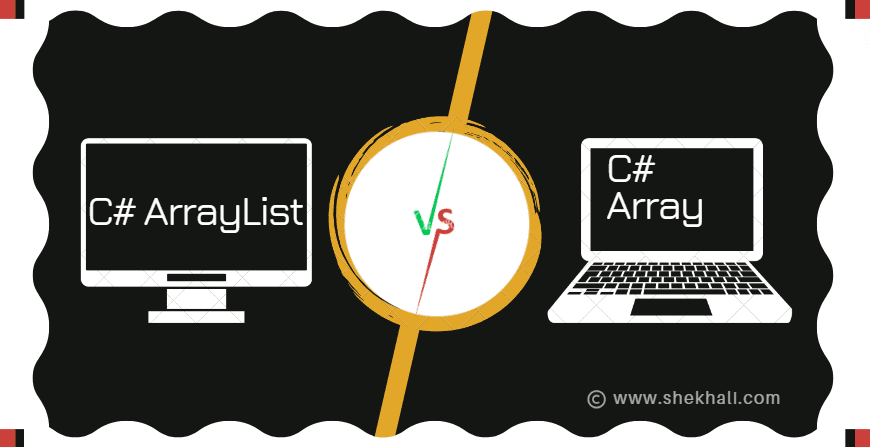
Table of Contents
C# Array
An array is a strongly type collection of elements of the same data type. It has a fixed length that cannot be changed during runtime.
Code Example: Declaring and Initializing Arrays
// Array of integers with a fixed size of 5
int[] numbersArray = new int[5] { 10, 20, 30, 40, 50 };
// Array of strings with a fixed size of 3
string[] namesArray = new string[3] { "Arjun", "John", "Putin" };
The new
keyword is used to allocate memory for the array, and the size is specified within square brackets []
. The Array elements are then initialized within curly braces {}
. Remember, you cannot add or remove elements from an array after its creation.
C# ArrayList
An ArrayList is not a strongly type collection. It can store values of different data types or the same data type.
The size of an ArrayList dynamically grows or shrinks based on the number of elements it contains.
This adaptability makes ArrayList ideal for scenarios where the number of elements is unknown or might change during the program’s execution.
Code Example: Declaring and Initializing ArrayLists
//Creating ArrayList to store objects of different types
ArrayList myList = new ArrayList();
// Adding elements using the Add method
myList.Add(100); // Integer
myList.Add("India"); // String
myList.Add(true); // Boolean
// Accessing elements using index (similar to arrays)
int firstElement = (int)myList[0]; // Casting required for type safety
// Displaying elements from ArrayList using a loop
foreach (object item in myList)
{
Console.WriteLine(item);
}
The ArrayList
class from the System.Collections
namespace is used to create an ArrayList.
Unlike arrays, you don’t need to specify the initial size. In ArrayList
, you can add elements of any data type using the Add
method. Accessing elements is done using an index, just like with arrays.
However, since ArrayLists store elements as objects, casting is often required for type safety when retrieving elements.
Understanding Array vs ArrayList in C#
Feature | Array | ArrayList |
---|---|---|
Size | Fixed-size: The size of an array is determined at declaration and cannot be changed during program execution. | Dynamic: ArrayLists can grow or shrink in size at runtime to adapt to your data needs. |
Data Type | Strongly typed: Arrays can only hold elements of a single, predefined data type (e.g., int[] , string[] ). | Non-generic: ArrayLists can store elements of any data type (mixed types are allowed). However, retrieving elements requires casting for type safety. |
Performance | Generally faster: Arrays offer direct memory access for elements, leading to better performance, especially for frequent access. | Slower: ArrayLists might incur some overhead due to potential boxing/unboxing operations when storing and retrieving elements of different data types. |
Thread Safety | Not thread-safe: Concurrent access to arrays from multiple threads can lead to data corruption if not properly synchronized. | Not thread-safe: Similar to arrays, ArrayLists are not thread-safe. For thread-safe collections in multithreaded scenarios, consider using the ConcurrentBag class. |
Common Methods | Limited built-in methods (e.g., Length , GetValue , SetValue ) for basic operations on elements. | Richer set of methods (e.g., Add , Remove , Sort , Search ) for easier manipulation and management of the collection. |
Namespace | System.Array | System.Collections |
Null Acceptance | Array cannot accept null. | ArrayLists accepts null. |
FAQs: C# Array vs ArrayList
Can I resize an array in C#?
No, the size of an array cannot be changed after it’s declared. If you need a dynamic collection, use an ArrayList or consider alternatives like List<T>
 from the System.Collections.Generic
 namespace, which offers generics for type safety and better performance.
When should I use an array, and when should I use an ArrayList?
Use an array when you need a fixed-size collection of elements of the same data type.
Use an ArrayList when you require a dynamic collection that can grow or shrink in size and can hold elements of any data type.
What is an ArrayList in C#?
An ArrayList
 is a dynamically resizable collection that can store values of different data types. It is not strongly typed and grows or shrinks based on the number of elements it contains.
Can an array store null values?
No, arrays cannot store null values. The default value for numeric array elements is zero, and for reference elements, it’s null.
How do I declare and initialize an array in C#?
You can declare and initialize an array as follows:int[] intArray = new int[] { 1, 2, 3 };
Here are some recommended articles on collections:
- C# Queue with examples
- C# Stack
- C# Dictionary
- C# Hashtable
- Collections in C#
- Array vs List
- C# List with examples
- C# Arrays
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024