If you are working with date and time values in C#, then it’s important to understand the DateTime
format in C#. DateTime is a built-in class in C# representing a date and time value. In this article, we will cover everything you need to know about the DateTime format in C#, including different formats and examples.
Table of Contents
- 1 C# DateTime Format
- 2 DateTime Formats using ToString() method
- 3 Custom DateTime formatting
- 4 C# DateTime Formatting Using String Interpolation
- 5 Convert Timestamp to Date and Date to Timestamp
- 6 FAQs
- 6.1 Q: How do you format DateTime in C#?
- 6.2 Q: What is the difference between d and D format in DateTime?
- 6.3 Q: How do you convert a string to DateTime in C#?
- 6.4 Q: Can you convert a Unix timestamp to a date in C#?
- 6.5 Q: What are the differences between the ToString() and the ToString(format) methods in C#?
- 6.6 Q: How can I get the current date and time in C#?
- 6.7 Related
C# DateTime Format
C# DateTime format is a set of codes that represents the date and time in a specific format. We can use these formats with the ToString() method to convert the DateTime object into a string.
The most commonly used format is “d”, which represents the short date pattern. The following code snippet shows an example of how to use this format:
DateTime now = DateTime.Now;
string shortDateFormat = now.ToString("d");
Console.WriteLine("Short Date Format: " + shortDateFormat);
Output: Short Date Format: 4/15/2023
The following are some of the standard DateTime formats in C#:
Format | Description | Example |
---|---|---|
d | Short date pattern | 4/15/2023 |
D | Long date pattern | Saturday, April 15, 2023 |
f | Full date/time pattern (short time) | Saturday, April 15, 2023 12:30 PM |
F | Full date/time pattern (long time) | Saturday, April 15, 2023 12:30:53 PM |
g | General date/time pattern (short time) | 4/15/2023 12:30 PM |
G | General date/time pattern (long time) | 4/15/2023 12:30:53 PM |
m | Month/day pattern | April 15 |
M | Month/day pattern | April 15 |
o | Round-trip date/time pattern | 2023-04-15T21:48:53.1234567-07:00 |
O | Round-trip date/time pattern | 2023-04-15T21:48:53.1234567-07:00 |
r | RFC1123 pattern | Sat, 15 Apr 2023 21:48:53 GMT |
R | RFC1123 pattern | Sat, 15 Apr 2023 21:48:53 GMT |
s | Sortable date/time pattern | 2023-04-15T12:30:00 |
t | Short time pattern | 12:30 PM |
T | Long time pattern | 12:30:00 PM |
DateTime Formats using ToString() method
Here is an example of how to use the ToString()
method in C# to format a DateTime value:
// Create a DateTime object
DateTime myDate = new DateTime(2023, 4, 15, 12, 30, 0, 0);
// Format the DateTime value using various standard format strings
Console.WriteLine(myDate.ToString("d")); // 4/15/2023
Console.WriteLine(myDate.ToString("D")); // Saturday, April 15, 2023
Console.WriteLine(myDate.ToString("t")); // 12:30 PM
Console.WriteLine(myDate.ToString("T")); // 12:30:00 PM
Console.WriteLine(myDate.ToString("f")); // Saturday, April 15, 2023 12:30 PM
Console.WriteLine(myDate.ToString("F")); // Saturday, April 15, 2023 12:30:00 PM
Console.WriteLine(myDate.ToString("g")); // 4/15/2023 12:30 PM
Console.WriteLine(myDate.ToString("G")); // 4/15/2023 12:30:00 PM
Console.WriteLine(myDate.ToString("M")); // April 15
Console.WriteLine(myDate.ToString("R")); // Sat, 15 Apr 2023 12:30:00 GMT
Console.WriteLine(myDate.ToString("s")); // 2023-04-15T12:30:00
Console.WriteLine(myDate.ToString("u")); // 2023-04-15 12:30:00Z
Console.WriteLine(myDate.ToString("U")); // Saturday, April 15, 2023 7:00:00 PM
Console.WriteLine(myDate.ToString("Y")); // April, 2023
Output:
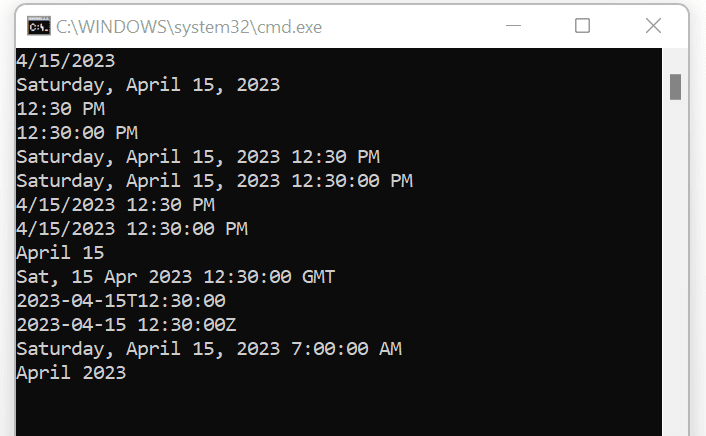
Custom DateTime formatting
When you want to display a date and time in a specific way that is not a standard pre-defined format, you can use a custom format string.
This format string is made up of special codes that tell the computer how to display the date and time.
Anything that is not a standard date and time format string is interpreted as a custom format string. These special codes are called format specifiers, allowing you to control the exact appearance of the date and time.
Here is an example of using custom date format specifiers with DateTime
values:
// Customt date and time formatting
//DateTime currentDate = DateTime.Now;
DateTime currentDate = new DateTime(2023, 4, 15, 12, 30, 0, 0);
Console.WriteLine(currentDate.ToString("MM/dd/yy")); // 04/15/23
Console.WriteLine(currentDate.ToString("MM/dd/yyyy")); // 04/15/2023
Console.WriteLine(currentDate.ToString("dd/MM/yy")); // 15/04/23
Console.WriteLine(currentDate.ToString("dd-MM-yy")); // 15-04-23
Console.WriteLine(currentDate.ToString("ddd, dd MMM yyyy")); // Sat, 15 Apr 2023
Console.WriteLine(currentDate.ToString("dddd, dd MMMM yy")); // Saturday, 15 April 23
Console.WriteLine(currentDate.ToString("dddd, dd MMMM yyyy HH:mm")); // Saturday, 15 April 2023 12:30
Console.WriteLine(currentDate.ToString("MM/dd/yy HH:mm")); // 04/15/23 12:30
Console.WriteLine(currentDate.ToString("MM/dd/yyyy hh:mm tt")); // 04/15/2023 12:30 PM
Console.WriteLine(currentDate.ToString("MM/dd/yyyy H:mm t")); // 04/15/2023 12:30 P
Console.WriteLine(currentDate.ToString("MM/dd/yyyy H:mm:ss")); // 04/15/2023 12:30:00
Console.WriteLine(currentDate.ToString("MMM dd")); // Apr 15
Console.WriteLine(currentDate.ToString("MM-dd-yyyyTHH:mm:ss.fff")); // 04-15-2023T12:30:00.000
Console.WriteLine(currentDate.ToString("MM-dd-yyyy g")); // 04-15-2023 A.D.
Console.WriteLine(currentDate.ToString("HH:mm")); // 12:30
Console.WriteLine(currentDate.ToString("hh:mm tt")); // 12:30 PM
Console.WriteLine(currentDate.ToString("HH:mm:ss")); // 12:30:00
Console.WriteLine(currentDate.ToString("'Full DateTime:' MM-dd-yyyyTHH:mm:ss")); // Full DateTime: 04-15-2023T12:30:00
C# DateTime Formatting Using String Interpolation
Here is an example of how to use String Interpolation in C# to format a DateTime
value:
// Get the current date and time
DateTime mydate = DateTime.Now;
// Day formats
Console.WriteLine($"\"d\" -> {mydate:d}"); // "d" -> 4/15/2023
Console.WriteLine($"\"d/M/yy\" -> {mydate:d/M/yy}"); // "d/M/yy" -> 15/4/23
Console.WriteLine($"\"dd\" -> {mydate:dd}"); // "dd" -> 15
Console.WriteLine($"\"ddd\" -> {mydate:ddd}"); // "ddd" -> Sat
Console.WriteLine($"\"dddd\" -> {mydate:dddd}"); // "dddd" -> Saturday
// Month formats
Console.WriteLine($"\"M\" -> {mydate:M}"); // "M" -> April 15
Console.WriteLine($"\"d/M/yy\" -> {mydate:d/M/yy}"); // "d/M/yy" -> 15/4/23
Console.WriteLine($"\"MM\" -> {mydate:MM}"); // "MM" -> 04
Console.WriteLine($"\"MMM\" -> {mydate:MMM}"); // "MMM" -> Apr
Console.WriteLine($"\"MMMM\" -> {mydate:MMMM}"); // "MMMM" -> April
// Year formats
Console.WriteLine($"\"y\" -> {mydate:y}"); // "y" -> April 2023
Console.WriteLine($"\"yy\" -> {mydate:yy}"); // "yy" -> 23
Console.WriteLine($"\"yyy\" -> {mydate:yyy}"); // "yyy" -> 2023
Console.WriteLine($"\"yyyy\" -> {mydate:yyyy}"); // "yyyy" -> 2023
Console.WriteLine($"\"yyyyy\" -> {mydate:yyyyy}"); // "yyyyy" -> 02023
// Hour formats
Console.WriteLine($"\"mm/dd/yy h\" -> {mydate:MM/dd/yy h}"); // "mm/dd/yy h" -> 04/15/23 10
Console.WriteLine($"\"hh\" -> {mydate:hh}"); // "hh" -> 10
Console.WriteLine($"\"mm/dd/yy H\" -> {mydate:MM/dd/yy H}"); // "mm/dd/yy H" -> 04/15/23 22
Console.WriteLine($"\"HH\" -> {mydate:HH}"); // "HH" -> 22
// Minute formats
Console.WriteLine($"\"m\" -> {mydate:m}"); // "m" -> 50April 15
Console.WriteLine($"\"mm\" -> {mydate:mm}"); // "mm" -> 27
Console.WriteLine($"\"h:m\" -> {mydate:h:m}"); // "h:m" -> 10:27
Console.WriteLine($"\"hh:mm\" -> {mydate:hh:mm}"); // "hh:mm" -> 10:27
// Second formats
Console.WriteLine($"\"s\" -> {mydate:s}"); // "s" -> 2023-04-15T22:27:15
Console.WriteLine($"\"ss\" -> {mydate:ss}"); // "ss" -> 15
// AM/PM
Console.WriteLine($"\"hh:mm t\" -> {mydate:hh:mm t}"); // "hh:mm t" -> 10:27 P
Console.WriteLine($"\"hh:mm tt\" -> {mydate:hh:mm tt}"); // "hh:mm tt" -> 10:27 PM
//timezone formats
Console.WriteLine($"\"mm/dd/yy K\" -> {mydate:MM/dd/yy K}"); "mm/dd/yy K" -> 04/15/23 +05:30
Console.WriteLine($"\"mm/dd/yy z\" -> {mydate:MM/dd/yy z}"); "mm/dd/yy z" -> 04/15/23 +5
Console.WriteLine($"\"zz\" -> {mydate:zz}"); "zz" -> +05
Console.WriteLine($"\"zzz\" -> {mydate:zzz}"); "zzz" -> +05:30
Convert Timestamp to Date and Date to Timestamp
Here is an example to convert date to timestamp and timestamp to date in C#
// Example: DateTime to Unix Timestamp and Vice versa.
// Converting Date to Timestamp
DateTime currentTime = DateTime.Now;
long timestamp = (long)(currentTime.Subtract(new DateTime(1970, 1, 1, 0, 0, 0))).TotalSeconds;
Console.WriteLine($"Converting DateTime {currentTime} to timestamp {timestamp}");
// Converting Timestamp to Date
DateTime dateTime = new DateTime(1970, 1, 1, 0, 0, 0).AddSeconds(timestamp);
Console.WriteLine($"Converting timestamp {timestamp} to Datetime: {dateTime}");
Output:
Converting DateTime 4/16/2023 12:44:42 AM to timestamp 1681605882
Converting timestamp 1681605882 to Datetime: 4/16/2023 12:44:42 AM
FAQs
Q: How do you format DateTime in C#?
We can use the ToString()
method with standard DateTime formats or custom date format specifiers to format DateTime values in C#.
Q: What is the difference between d and D format in DateTime?
The “d” format represents the short date pattern, while the “D” format represents the long date pattern.
Q: How do you convert a string to DateTime in C#?
We can use DateTime.Parse()
or DateTime.TryParse()
method to convert a string to DateTime in C#.
Q: Can you convert a Unix timestamp to a date in C#?
Yes, we can convert a Unix timestamp to a date in C# by using the DateTimeOffset.FromUnixTimeSeconds()
method.
Q: What are the differences between the ToString() and the ToString(format) methods in C#?
The ToString()
method is used to convert a DateTime object to its default string representation. The ToString(format)
method, on the other hand, is used to convert a DateTime object to a string representation that conforms to a specific format.
Q: How can I get the current date and time in C#?
You can get the current date and time in C# using the DateTime.Now
property.
Check this Online tool to Convert timestamp to date and date to timestamp
Recommended Articles:
- Format String in C# with examples
- Interface in C# with examples
- IEnumerable Vs IQueryable
- Singleton Design Pattern in C#: A Beginner’s Guide with Examples
- Array Vs List in C#
- C# Collection with code examples
- C# Dependency Injection with example
- SOLID Design Principles in C#: A Complete Example
- Difference between interface and abstract class In C#
- C++ vs C#: Difference Between C# and C++
- C# Regex: Introduction to Regular Expressions in C# with Examples
- C# String Interpolation – Syntax, Examples, and Performance
- C# Stopwatch Explained – How to Measure Code Execution Time?
- C# String Vs StringBuilder
- C# Queue with examples
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024