Welcome to our comprehensive C# tutorial, where you will dive into this powerful programming language’s fundamental and advanced concepts. Whether you are a complete beginner or a seasoned professional, our C# tutorial is designed to cater to all levels of expertise.
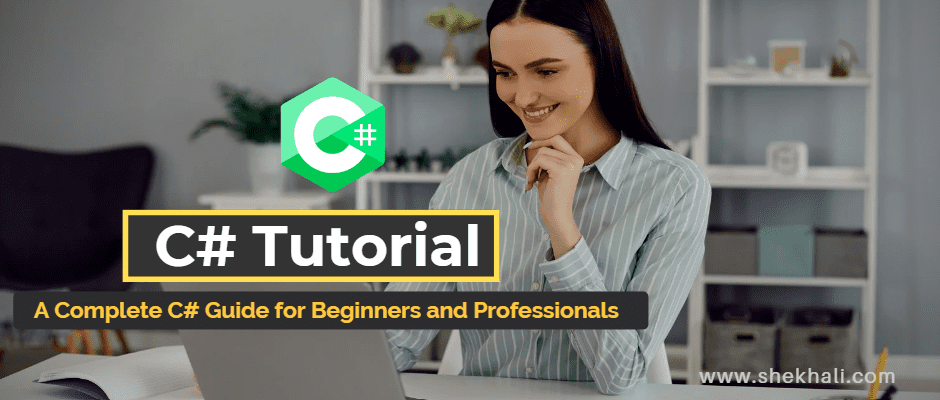
C# Fundamentals
- Understanding C# Class and Objects
- C# Struct with examples
- Understanding C# Abstract Class
- Interface in C#
- Encapsulation in C#
- Polymorphism in C#
- Enum Type in C#
- String vs StringBuilder in C#
- Anonymous method in C#
- Value Type and Reference Type in C#
- Partial class and methods in C#
- Extension methods in C#
C# Conditions & Loops
C# Collections
C# Articles
- Garbage Collection in C# .NET
- Difference Between Overriding and Overloading in C#
- Understanding List vs Dictionary in C# With Examples
- C# dispose vs finalize in C# .NET
- Static vs Singleton in C#: Understanding the Key Differences
- Understanding 3-Tier Architecture in C# .NET
- Understanding Covariance And Contravariance in C#
- C# Struct vs Class
- C# Interface vs Abstract class
- DateTime Format in C#
- Inheritance in C#
- Hybrid Inheritance in C#
- Difference between abstraction and encapsulation in C#
- Class vs Struct vs Record in C#
- C# Static Class
- C# Lock vs Mutex
- Python vs C#
- C++ vs C#
- Ienumerable vs Iqueryable Interface
- C# Hashtable vs Dictionary vs Hashset
- Is vs AS Operator in C#
- Difference between SortedList and SortedDictionary
- Equality Operator (==) vs Equals method in C#
- Stack vs Heap memory
- Boxing vs Unboxing in C#
- Delegates in C# with examples
- Generic delegates in C# with examples
- C# Early Binding vs Late Binding
- C# Tuple with examples
- Understanding Ref and Out keywords in C#
- Difference between Var and Dynamic in C#
- Var keyword in C#
- Types of Constructor in C#
- Copy Constructor in C#
- Static Constructor in C#
- Private Constructor in C#
- Sealed class in C#
- How to check if string is null or empty
- C# TextWriter
- C# Regex example
- Format string in C#
- Difference between Int, Int16, Int32, and Int64
- Understanding C# Reflection
- C# StopWatch Class
- C# String Interpolation
- Understanding C# 10 new features
- Understanding Non-Generic Collections in C#
- Indexer in C#
- Generics in C#
- Difference between field and property in C#
- Properties in C#
- Understanding Access modifiers in C#
- Read-Only vs Constant in C#
- Understanding GoTo statement in C#
- Understanding System.IO Classes
- Local vs Global variable in C#
- Multithreading in C# with examples
- Constant vs Static vs ReadOnly in C#
- Try Catch block in Exception Handling
- Top 50 C# Interview Questions and Answers
- C# Param keyword
- C# Nullable types
- C# Dynamic Type with examples
- Understanding C# Unsafe Code
- C# Events with examples
- C# StreamReader and StreamWriter
- IEnumerable Interface in C#
- Types of Comments in C#