Enhance your coding skills in C# with this comprehensive list of programming examples.
Here, we cover basic topics such as arrays, strings, control statements, etc. Below is the list of C# programs frequently asked in interviews.
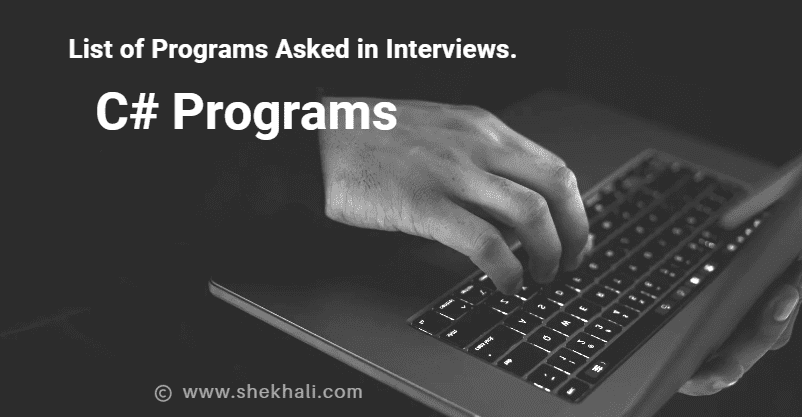
C# Basic Programs
- Fibonacci sequence: Fibonacci series in C# (with examples)
- Reverse a number and string in C#
- C# Program to Find the Longest Word in a String
- C# Program to capitalize the first character of each word in a string
- How to remove duplicate characters from a String in C#
- C# Program to Check if a Given Number is Even or Odd
- C# program to count the occurrences of each character in a String
- C# program to count vowels and consonants in a string
- Palindrome program in C# with examples
- C# Program to Print Multiplication Table of a Given Number
- How to remove special characters from a string in C#
- C# Program to swap two numbers
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- Leap Year Program in C, C++, C#, JAVA, PYTHON, and PHP
- Program to print prime numbers from 1 to N
- Bubble sort programs in C, C++, JAVA, and PYTHON
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- C# Program to Check Armstrong Number
List Of Conversion Programs in C#
Array Programs in C#
- Program to copy all elements of an array into another array
- How to Reverse an Array in C# with Examples
- Program To Find Largest Number In An Array
- Remove Duplicate Elements from an Array
- Convert a 2D array into a 1D array
- C# program to find the maximum and minimum number in an array
- Find the missing number in an array
- C# Program to rotate an array by k position
- C# program to find all duplicate elements in an integer array