Table of Contents
Problem Statement:
Consider a scenario where you have a string and need to capitalize the first letter of each word in that string. For example, if the input string is “hello world,” the output should be “Hello World.”
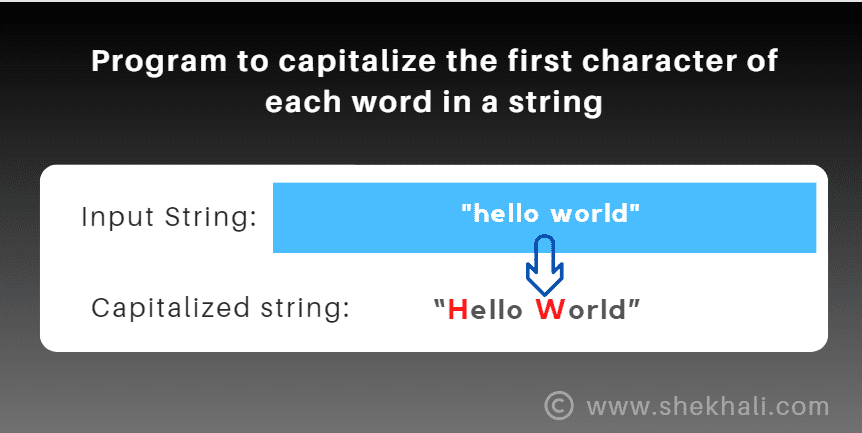
Solution Approach:
We’ll break down the problem into smaller steps and write a C# Program to Capitalize the first character of each word in a String.
Method 1: Capitalize the First Character of Each Word Using LINQ
We can split the input string into words, capitalize the first character of each word, and then join them back into a string. Here’s how we can achieve this using LINQ:
using System;
using System.Linq;
namespace CapitalizeWords
{
class Program
{
/* C# Program to Capitalize a First letter of Each word in a string*/
static void Main(string[] args)
{
string inputString = "hello world";
Console.WriteLine($"Original string: { inputString}");
Console.WriteLine($" Capitalized string: {CapitalizeWords(inputString)}");
}
public static string CapitalizeWords(string inputString)
{
// First, Split the input string into words and then capitalize the first character of each word,
// and join them back
return string.Join(" ", inputString.Split(' ').Select(word => char.ToUpper(word[0]) + word.Substring(1)));
}
}
}
Output:
Original string: hello world
Capitalized string: Hello World
Method 2: Using TextInfo.ToTitleCase()
Another approach is to use the TextInfo.ToTitleCase()
method from the System.Globalization
namespace. This method capitalizes the first character of each word in a given string:
using System;
using System.Globalization;
namespace CapitalizeWords
{
class Program
{
/* Program to Capitalize a First letter of Each word in a string in C# */
static void Main(string[] args)
{
string inputString = "hello world";
Console.WriteLine($"Original string: { inputString}");
string outputString = CultureInfo.CurrentCulture.TextInfo.ToTitleCase(inputString.ToLower());
Console.WriteLine($"Capitalized string: { outputString}");
}
}
}
Output:
Original string: hello world
Capitalized string: Hello World
Method 3: Using String Manipulation Functions
Let’s write a C# Program to capitalize the first character of each word in a string using manipulation functions.
using System;
class Program
{
/* C# Program to Capitalize a First letter of Each word in a string*/
static void Main(string[] args)
{
string input = "hello world";
Console.WriteLine($"Original string:{input}");
string[] words = input.Split(' ');
for (int i = 0; i < words.Length; i++)
{
words[i] = char.ToUpper(words[i][0]) + words[i].Substring(1);
}
string output = string.Join(" ", words);
Console.WriteLine($"Capitalized string:{output}");
// Output: Hello World
}
}
Output:
Original string: hello world
Capitalized string: Hello World
Code Explanation:
- We split the input string into an array of words using the
Split
function. - Then, we iterate over each word and capitalize its first character using
char.ToUpper
and string concatenation. - Finally, we join the modified words back into a string using
string.Join
.
Method 4: Using Regular Expressions
Now we will write a C# Program to capitalize the first character of each word in a string using regular expressions.
using System;
using System.Text.RegularExpressions;
class Program
{
static void Main(string[] args)
{
string input = "hello world";
string output = Regex.Replace(input, @"\b\w", m => m.Value.ToUpper());
Console.WriteLine(output); // Output: Hello World
}
}
Code Explanation:
- In the above code snippet, We have used a regular expression
\b\w
to match the first character of each word. - The
Regex.Replace
function replaces each match with its uppercase equivalent.
Recommended Articles:
- Find the maximum and minimum number in an array
- How to Reverse an Array in C# with Examples
- Program to copy all elements of an array into another array
- C# Program to Convert Decimal to Binary with Examples
- C# Program to Convert Binary to Decimal with Examples
- C# Program to Check Armstrong Number
- How to remove duplicate characters from a String in C#
- C# program to count the occurrences of each character in a String
- C# Program to Print Multiplication Table of a Given Number
- Program to print prime numbers from 1 to N
- C# Program to Check if a Given Number is Even or Odd
- Program to copy all elements of an array into another array
- Different Ways to Calculate Factorial in C# (with Full Code Examples)
- C# Programs asked in Interviews
- Difference Between Array And ArrayList In C#: Choosing the Right Collection - May 28, 2024
- C# Program to Capitalize the First Character of Each Word in a String - February 21, 2024
- C# Program to Find the Longest Word in a String - February 19, 2024